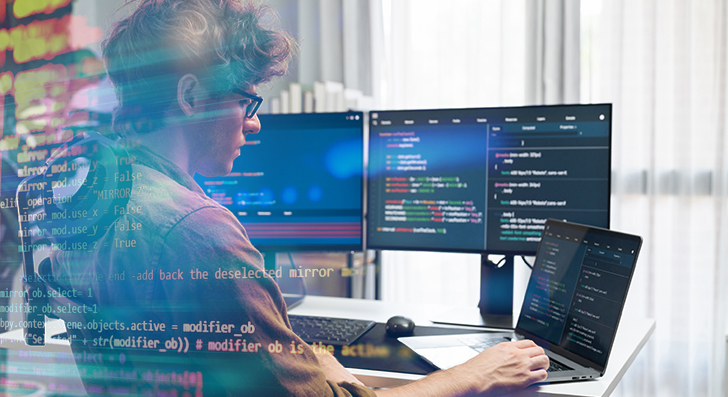
Scalability implies your application can manage growth—extra people, far more information, and much more targeted visitors—devoid of breaking. Being a developer, developing with scalability in your mind saves time and worry later on. Right here’s a transparent and simple guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be part of your respective strategy from the start. Numerous apps fail if they develop rapid simply because the original layout can’t handle the extra load. To be a developer, you should Imagine early about how your technique will behave stressed.
Start off by designing your architecture to get adaptable. Steer clear of monolithic codebases wherever everything is tightly linked. As a substitute, use modular style or microservices. These designs break your app into more compact, unbiased parts. Each and every module or assistance can scale By itself with out impacting The full method.
Also, contemplate your databases from working day one. Will it want to manage one million buyers or simply a hundred? Pick the ideal type—relational or NoSQL—depending on how your info will increase. System for sharding, indexing, and backups early, Even when you don’t want them however.
Yet another important stage is to prevent hardcoding assumptions. Don’t compose code that only performs underneath latest disorders. Think about what would happen if your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use structure styles that aid scaling, like information queues or celebration-pushed units. These assistance your application cope with additional requests with no receiving overloaded.
If you Construct with scalability in mind, you're not just preparing for success—you might be cutting down foreseeable future head aches. A nicely-planned procedure is less complicated to keep up, adapt, and develop. It’s far better to organize early than to rebuild afterwards.
Use the appropriate Database
Choosing the right databases is actually a important part of building scalable purposes. Not all databases are created the identical, and utilizing the Erroneous one can gradual you down as well as trigger failures as your application grows.
Start off by comprehension your information. Can it be hugely structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an effective fit. These are definitely sturdy with associations, transactions, and regularity. Additionally they aid scaling tactics like read replicas, indexing, and partitioning to manage more website traffic and information.
If the info is more versatile—like person action logs, products catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling huge volumes of unstructured or semi-structured details and might scale horizontally more very easily.
Also, take into consideration your study and produce styles. Have you been accomplishing plenty of reads with less writes? Use caching and browse replicas. Are you dealing with a major create load? Investigate databases which can handle large produce throughput, or simply occasion-based mostly knowledge storage units like Apache Kafka (for temporary info streams).
It’s also sensible to Assume in advance. You might not need Sophisticated scaling characteristics now, but picking a databases that supports them suggests you won’t want to change later on.
Use indexing to speed up queries. Keep away from unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And normally monitor database efficiency while you expand.
In a nutshell, the correct database is determined by your app’s structure, speed needs, And exactly how you anticipate it to develop. Consider time to pick sensibly—it’ll help you save many issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, every compact hold off adds up. Poorly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Construct efficient logic from the beginning.
Start off by producing clear, straightforward code. Steer clear of repeating logic and take away everything needless. Don’t choose the most advanced Resolution if a simple a person performs. Maintain your functions shorter, targeted, and easy to check. Use profiling tools to uncover bottlenecks—spots where your code can take also long to operate or employs an excessive amount of memory.
Upcoming, examine your databases queries. These usually gradual items down much more than the code by itself. Be certain Each and every question only asks for the data you really need to have. Avoid Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specifically throughout large tables.
For those who discover the exact same data getting asked for again and again, use caching. Retailer the final results quickly utilizing equipment like Redis or Memcached this means you don’t need to repeat high-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your app additional economical.
Make sure to test with huge datasets. Code and queries that operate fine with 100 information may well crash if they have to take care of one million.
To put it briefly, scalable applications are fast applications. Keep the code tight, your queries lean, and use caching when needed. These actions enable your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage far more end users plus much more website traffic. If anything goes by just one server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available. Both of these instruments support maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As opposed to just one server executing every one of the perform, the load balancer routes customers to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent alternatives from AWS and Google Cloud make this very easy to setup.
Caching is about storing data briefly so it may be reused immediately. When people request a similar facts once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database every time. You may serve it within the cache.
There are 2 common forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for quick entry.
2. Customer-side caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust often. And often be certain your cache is up to date when data does adjust.
To put it briefly, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app manage additional users, remain rapid, and recover from difficulties. If you intend to mature, you may need both of those.
Use Cloud and Container Tools
To create scalable more info apps, you would like tools that let your app increase conveniently. That’s where cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t have to purchase hardware or guess potential capability. When site visitors will increase, it is possible to insert additional methods with just a couple clicks or mechanically using auto-scaling. When traffic drops, you are able to scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and stability applications. You could center on making your application as opposed to handling infrastructure.
Containers are An additional key Software. A container offers your app and almost everything it should run—code, libraries, configurations—into just one device. This makes it easy to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs numerous containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You could update or scale elements independently, which is perfect for performance and dependability.
In short, using cloud and container instruments indicates you may scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to mature without having restrictions, begin working with these tools early. They preserve time, cut down danger, and make it easier to stay centered on building, not fixing.
Keep an eye on Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Checking helps you see how your app is doing, location issues early, and make much better choices as your application grows. It’s a critical part of developing scalable devices.
Start by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just keep an eye on your servers—watch your application far too. Regulate how much time it's going to take for buyers to load internet pages, how frequently faults occur, and in which they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for important problems. For example, if your reaction time goes higher than a Restrict or possibly a provider goes down, you ought to get notified right away. This assists you repair issues speedy, generally in advance of end users even recognize.
Monitoring can also be useful after you make improvements. In case you deploy a fresh feature and find out a spike in problems or slowdowns, you are able to roll it again in advance of it triggers real destruction.
As your app grows, visitors and details enhance. With out checking, you’ll overlook signs of difficulties until finally it’s much too late. But with the best resources in place, you keep in control.
Briefly, monitoring can help you keep your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it works perfectly, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even compact apps will need a strong Basis. By designing meticulously, optimizing sensibly, and using the suitable equipment, you could Construct applications that mature easily devoid of breaking under pressure. Commence smaller, think huge, and Establish intelligent.